Problem
Given a generic iOS application ‘Blog’ with data model as below:
We want to prepopulate data for ‘Categories’ table in our SQLite database.
Solution
This solution uses the approach described in Core Data on iOS 5 Tutorial: How To Preload and Import Existing Data, we will create an OS X Command Line Tool application, which will reference the data model in our ‘Blog’ application and prepopulate the data. Except we automate the building process.
Step 1
Make sure you are using Xcode Workspace with your project and skip to Step 2. If not, follow the steps below to create a new workspace:
- Open your project in Xcode
- Goto File > New > Workspace… or use the shortcut Command + Control + N
- Name the new workspace the same as your project and save it in your project folder.
- Quit Xcode
- Open the Workspace
$ open Blog.xcworkspace
- Drag your project from Finder to the Workspace
- We have successfully added your project into a workspace.
Step 2
- While your workspace is open create a new project by selecting File > New > Project
- From the prompt window select OS X > Application > Command Line Tool and click Next
- In the next window enter MasterDataLoader for the Product Name, select Core Data in the Type pulldown and click Next
- In the next window select the folder where your workspace is stored, select your workspace for Add to and Group pulldowns. Click Create.
- Now you have two applications in your workspace
- Remove the MasterDataLoader.xcdatamodeld file from the MasterDataLoader application and Move to Trash
- Open the
main.m
file in the MasterDataLoader application - Change the lines in
managedObjectModel()
andmanagedObjectContext()
methods from
1 2 |
|
1 2 |
|
to
1
|
|
Now run MasterDataLoader application.
You should get an error Cannot create an NSPersistentStoreCoordinator with a nil model
.
Now we made sure our command line app runs and can’t find a compiled data model object. Great!
Step 3
Add a new Run Script build phase to your ‘Blog’ application, name it Run MasterDataLoader and drag it under Compile Sources phase.
Now paste this code in the run script you just created and save.
1 2 3 4 5 6 7 8 |
|
Edit the scheme for ‘Blog’ application. Add MasterDataLoader in build targets.
This step makes sure that MasterDataLoader is build before the ‘Blog’ app.
Step 4
Open AppDelegate.m
in the ‘Blog’ application and add the following code
1 2 3 4 5 6 7 |
|
under the line
1
|
|
As you can see, here we check if the SQLite database already exists. If not, we copy the one which is in the main bundle to the ‘Documents’ folder.
Right now our SQLite datamase is empty. Let’s fetch ‘Categories’ and check if the table is empty.
Add the following code to the application:didFinishLaunchingWithOptions:
method just before return YES
line.
1 2 3 4 5 6 7 8 |
|
Don’t forget to import Category.h
1
|
|
Run the ‘Blog’ application. The console should print nothing.
Step 5
Lets add some Categories
.
Drag the Category.h
file from ‘Blog’ application to MasterDataLoader application.
Open the main.m
file. Import Category.h
.
1
|
|
Replace the code in main()
method
1 2 3 4 5 6 7 |
|
with
1 2 3 4 5 6 7 8 9 |
|
Before running
Delete ‘Blog’ application from the simulator.
Run
Run the ‘Blog’ application.
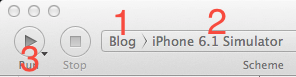
The console should print three categories:
1 2 3 |
|
Discussion
This way you can reference any managed object from MasterDataLoader app and preload the data.